WebAssembly - An Overview
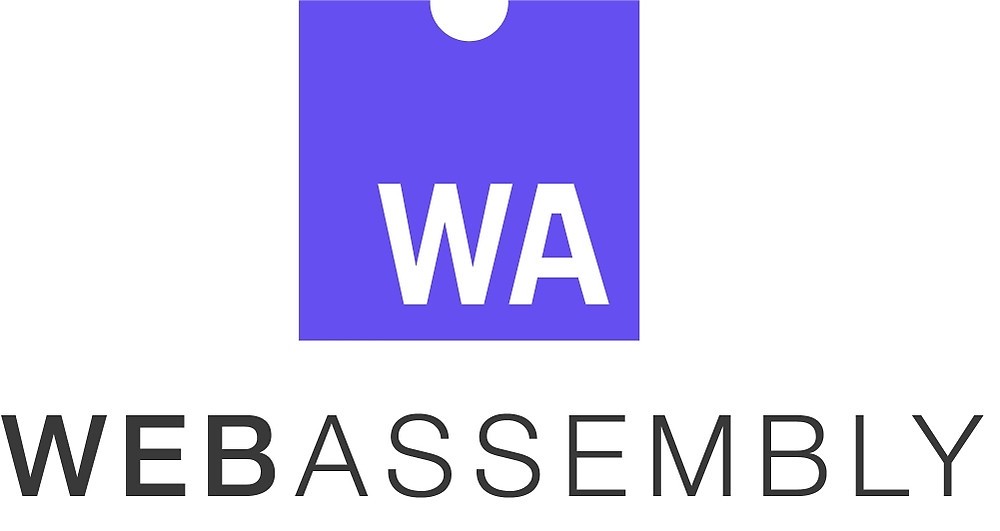
During one of my interviews recently (I got the job!) I had to give a presentation on a topic I was interested in. I ended up doing a presentation on WebAssembly! I’ve been working with web applications for the past couple of years so WebAssembly was an advancement I was excited for. This post is about what I learned about WebAssembly and why I think it’s an exciting addition to the web.
What is it?
If you want the formal definition you’re best off looking at the official WebAssembly website. Wasm is a new alternative to Javascript for the web where Wasm is treated like a new compiler target rather than a language you’d actually write your code in (though, you can do that, if that’s your idea of fun). While allowing developers to create web applications in programming languages other than Javascript it also brings with it a host of performance improvements too.
A First Class Alternative to Javascript
We’ve tried getting away from Javascript before, you’ll remember these:
- Flash
- Silverlight
- Java applets (the university I went to loved teaching us about applets - probably so they didn’t have to teach us Javascript…)
It’s pretty clear that embedding runtimes into the browser hasn’t worked in the long term. The decision by Apple on mobile to not include plugins like Flash definitely had an impact while security issues were the other problem.
Conversely, WebAssembly is not a plugin that has to be installed. It can run in the same sandboxed virtual machine as Javascript inheriting the safety and security of those virtual machines too. Better yet - it’s already supported (though not always optimised) in the major browsers and has been since 2017.
I’ll be using ‘Wasm’ a lot (although, somewhat inconsistently) in this post, I and many others also say it out loud too. It rhymes with spasm. 😁
The Origin of Wasm
I think it’s interesting to understand where Wasm came from. You may have heard of asm.js which was a POC by Mozilla back in 2013. asm.js is a strict subset of Javascript that can be used as a low-level compile target from languages like C and C++, the main benefit of asm.js is that it allows ahead of time compilation in Javascript. Ahead of time compilation in Javascript!?! How it manages to do this is pretty interesting.
Here’s a function that raises a number to a power in C
float power(float number, int pow) {
float res = number;
for (int i = 0; i < pow - 1; i++) {
res = res * number;
}
return res;
}
Here’s the equivalent code compiled in asm.js:
"use asm";
function X(a,b) {
a = +a;
b = b | 0;
var c = 0.0, d = 0;
d = b + -1 | 0;
if ((b | 0) > 1) {
b = 0;
c = a;
} else return +a;
do {
c = c * a;
b = b + 1 | 0;
} while ((b | 0) != (d | 0));
return +c;
}
You’ll notice that the above Javascript doesn’t look much like the Javascript you or I would write. What makes the Javascript above different to ’normal’ Javascript is that it subtly includes type information. An explanation below:
a = +a; //enforces a number type +"1" -> 1
b = b | 0; //bitwise operation that always results in an integer, 2.4 | 0 -> 2
//try them out in your browsers console!
And there you go… Strongly typed Javascript. It’s ingenious.
The Next Step… Wasm
Wasm is similar to asm.js in a number of ways, except it’s no longer Javascript which means we no longer have the same limitations as Javascript. asm.js was the experiment, Wasm is the real thing.
Wasm is:
- A binary format (smaller download size than minified JS + faster to parse)
- Has four numerical types (2 integer based types and 2 floats)
- Backwards compatible with asm.js
and supports a wide variety of languages (not an exhaustive list):
- C/C++
- Rust
- Typescript (via AssemblyScript)
- C#
- Java
- (pretty much any strongly typed language)
You can even write WebAssembly in Javascript-like syntax with Walt.
Replace Javascript?
The stated goal is not to replace Javascript but to ‘complement’ it. Very diplomatic.
In the short term, it obviously won’t. In the long term, I don’t think so either. Javascript is in quite a good place currently with all the various frameworks available like React. I’m sure there are people out there that enjoy writing Javascript more than they do writing languages like C# or Java. Look at the popularity of NodeJS - unlike in the browser writing Javascript on the server is a choice and a popular one at that. On that point, Wasm is available in NodeJS too…
There’s definitely been a need for types in web development though, this is where Typescript and other transpilers have come in. Wasm will instead be able to fill this gap and we will no longer have any need for transpilers, we don’t need to use Javascript like an assembly language anymore - something it was never meant to be.
It’ll be interesting to see in the next decade how much of a shift there is in the industry from Javascript to Wasm.
Wasm Features
Streaming Compilation
Streaming compilation is the ability to compile something while it’s downloading at the same time. This isn’t possible with Javascript, although Javascript can be parsed while downloading and it wouldn’t necessarily be compiled straight away anyway. Javascript generally only compiles code that gets used and isn’t compiled up front. Wasm, rather, must all be compiled up front. You’d think this upfront compilation would be an overhead compared to Javascript but thanks to streaming compilation it isn’t.
The way browsers are optimising this is by using tiered compilers. You might first have a compiler that compiles very quickly but doesn’t produce code that is very optimised and then a second compiler that takes significantly longer while producing more optimised code. By doing this Firefox is able to compile code faster than it can be downloaded on desktop CPU’s - soon after the download is done so is the compilation.
Background Compilation
What helps with tiered compilation is being able to compile in the background. Wasm can be compiled in background threads (across multiple threads at once) and when the compilation is done the newer optimised code can be hot-swapped on to the main thread.
Compilation within Javascript, on the other hand, all happens on the main thread. Compiling in Javascript sacrifices the current moment for better future performance. Javascript has always been about single core performance while Wasm scales across cores much better during load times which will help on mobile devices.
Benchmarks
Theory is great, but real-world use cases are better. Unity has written a good blog post with benchmarks on using Wasm with Unity with lots of nice graphs. I’ve included one below showing ’time to screen’ in Wasm vs asm.
To summarise Unity’s findings:
- Wasm works best with Firefox (it’s their baby after all)
- Load times are better with Wasm
- Execution speed improves across all browsers compared to asm.js
- Tiered compilation improved loading times massively
For Unity, everything is very positive indeed. Wasm pushes things forward a bit further for computationally intensive applications like VR, AI, Computer Vision and games on the web.
PSPDFKit also have a blog post about their benchmarks by moving PDF rendering logic from the server on to the client. You’ll see in the graph below that Wasm hasn’t had much of an effect unless you’re using Firefox.
It should be noted that PSPDFKit disabled one of the main advantages of Wasm in these benchmarks: streaming compilation. The reason they did this was because Javascript can’t stream and compile and they wanted to remove network speed noise from the equation. I’m not too convinced by the reasoning - Javascript only compiles small chunks of code while Wasm has to compile everything handicapping Wasm during load time. This does mean the benchmarks are weighted more in terms of execution speed rather than the overall picture though.
Execution Speed
Wasm in a way has been overly sold in terms of its execution speed. Articles with titles like “WASM is 84 times faster than JavaScript” have hyped Wasm up into something it isn’t.
One of the aims of Wasm is to produce code that runs almost as fast as native code. There was a paper released in the last few weeks titled Mind the Gap: Analyzing the Performance of WebAssembly vs Native Code by Abhinav Jangda, Bobby Powers et al. that studies this in thorough detail. They’ve found Wasm to be 2 or 3x slower in peak situations while on average it’s between 1.4x and 1.9x slower than the equivalent code in C depending on whether you’re using Firefox or Chrome.
I don’t think that kind of slowdown is that bad. But hey, maybe I’m completely wrong. From a lot of the benchmarks I’ve seen so far Wasm generally performs at least as good as Javascript, sometimes much better and sometimes there doesn’t appear to be any difference at all. There’s an occasional benchmark where it performs worse. Let’s not forget, Javascript isn’t slow - though it once was. Wasm is still very new and is yet to have been pushed with tons of optimisations like Javascript has been.
Caching
Once a Wasm module has been compiled it can be cached and reused later. This isn’t just caching the Wasm file but caching the compiled version - no downloading, no parsing, no compiling, nothing (aside from loading it from a cache). Firefox and Edge support this right now while it’s turned off by default in Chrome. Safari is lagging behind without any implementation for this so far.
Multi-threading
We can execute code in the background currently by using web workers, the limitation with them right now is that they are very independent. They’re more like different processes than threads. The only way to communicate between them is with postMessage at the moment.
With Wasm, web workers will be able to share memory between them, behaving a lot like how you would expect threads to behave in a traditional application (including managing access to that memory with locks).
Not Just for the Web
Given the name ‘WebAssembly’ you’d think it’s only useful for the web but you’d be wrong. There’s a lot of talk of WebAssembly becoming the new default intermediate representation that can run across all the major desktop and mobile operating systems.
Think of it as like Java bytecode that can run on any JVM, except with Wasm we’re not forced into using Java but can use pretty much any other strongly typed language we want. There are already independent Wasm virtual machines that exist outside of the browser like Life.
Even for duck-typed languages like Python, this can still work by compiling Python’s interpreter into Wasm and then running the Python code through that. Sure it’d be slow, but hey, nobody writes Python for performance.
Limitations
Garbage Collection
Currently, there’s no garbage collection at all. This limits Wasm in practical terms to those languages like C that don’t require a garbage collector. There are plans to add a garbage collector in the future.
No Direct DOM Access
If you want to access the DOM you have to do this indirectly through Javascript at the moment. There used to be a lot of concern over calls between Wasm and Javascript because they used to be very slow, but Firefox has changed that.
A lot of people using Wasm in production currently have a ‘view’ layer written in Javascript while Wasm acts as the business logic or some algorithm that needs to be performant.
There are plans for DOM access in the future but this needs garbage collection first.
Debugging
Wasm is a pain to debug. While Wasm is a binary format it does also come in a text-based format which you can open up in the browser dev tools.
Here’s that raising to a power function you saw earlier in Wasm’s text format:
(module
(table 0 anyfunc)
(memory $0 1)
(export "memory" (memory $0))
(export "_Z5powerfi" (func $_Z5powerfi))
(func $_Z5powerfi (; 0 ;) (param $0 f32) (param $1 i32) (result f32)
(local $2 f32)
(block $label$0
(br_if $label$0
(i32.lt_s
(get_local $1)
(i32.const 2)
)
)
(set_local $1
(i32.add
(get_local $1)
(i32.const -1)
)
)
(set_local $2
(get_local $0)
)
(loop $label$1
(set_local $2
(f32.mul
(get_local $2)
(get_local $0)
)
)
(br_if $label$1
(tee_local $1
(i32.add
(get_local $1)
(i32.const -1)
)
)
)
)
(return
(get_local $2)
)
)
(get_local $0)
)
)
… Ew. The typical strategy right now when debugging Wasm is to compile an asm.js version and then debug that. Long term, source maps will be the solution.
Yet More Frameworks Incoming…
I’m really excited about all of the new frameworks that will (and already are) coming along because of Wasm. I’ve used both AngularJS and Angular and I have to admit I prefer the latter mostly because of Typescript. I really like AngularJS too, but the JS part is something I find annoying. Not knowing what kind of model a service returns is frequently frustrating for example. Typescript is better - but it’s still not quite on the level of something like C#.
It’s crazy that given how long the web has been around the only way to run applications on the client up until recently was with Javascript. Wasm finally opens up the web to development in other languages.
Some examples of new frameworks that are in varying stages of development:
- Blazor (C#) - Razor style component based framework
- Ooui (C#) - an alternative to Blazor
- Flavour - TeaVM (Java) - JSTL style component based framework
- Yew (Rust)
- there’s lots more than this…
Blazor
Blazor is one of the most exciting and publicised frameworks that work with Wasm currently. I’ve given Blazor a try and I really recommend you do too - it’s really easy to get a project setup. It’s very similar to Angular in terms of its structure except it’s written in C# and .NET. You have the full .NET standard 2.0 API available to you (at build time at least). I tried implementing threads to see what would happen in the browser, all I got was a NotSupportedException sadly.
With a bit more work even threading could be compatible with Blazor. TeaVM who make a similar framework called Flavour can translate multithreaded apps into asynchronous versions. We’ll soon be reaching a point where you won’t need to make any specific changes to your code to make it suitable for the web.
The thing I love about Blazor is that it is proper .NET. There are no limitations when writing and building your code at all. I’ve worked with transpilers previously in C# and Java and they both had their limitations. I eventually got a Java physics engine working in Javascript but only after I modified a lot of code to get it to compile. That’s not an issue with Blazor. Sharing code between client and server is a nice benefit too.
Although Blazor compiles into Wasm don’t mistakenly believe it’s going to be fast - it’s not. Blazor currently runs a mono interpreter in Wasm and then downloads the DLL files for your projects and interprets the IL (intermediate language). To be fair, the Blazor team haven’t focused on optimisations at all yet, it’s all been about framework features to show off rather than speed.
Mono does have a prototype using ahead of time compilation with Blazor which compiles all your code into one big .wasm file instead of downloading DLLs which is likely going to be the end solution. Blazor will get faster.
Mono
The mono interpreter that compiles down to Wasm is a completely separate project from Blazor, Blazor just happens to use it. They’re not the only ones though.
Ooui is another project that’s making use of this and is similarly a new UI framework using .NET for Wasm. With Ooui you can even write Xamarin.Forms apps for the web with XAML. It, like Blazor, also looks really cool.
Conclusion
The future of Wasm is looking very exciting, and not just for the web. Wasm seems to be taking a big chunk of that ultimate goal of ‘write once, run anywhere’ that we never quite reach. Maybe we will this time?
Hopefully, you’ve learned something in this post, I’m not very experienced with ‘proper’ blogging (my previous blogs have been more like twitter feeds than blogs) so I suspect some of what I’ve written is unnecessary or unstructured. Ah well.