Domain Models
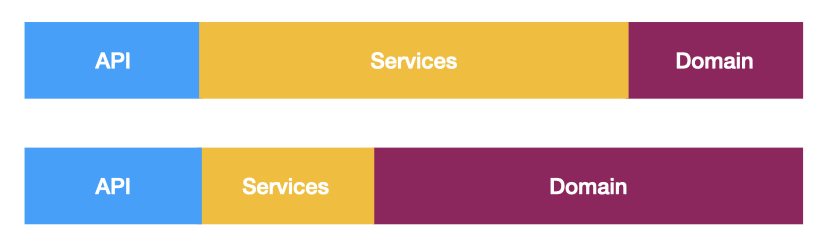
I wrote a post about dependency injection overuse back in April 2019 and I ended it with:
Dependencies don’t always need to or should be injected, sometimes using the
new
keyword is okay. Knowing what to inject and what not to inject is something I can’t quite define concretely right now. What I am certain of is that not every class you create needs the suffix ‘Service/Helper’ or needs to be registered in your container as a singleton each and every time.
This is less of a post about dependency injection but rather the issue I had with every type in the codebase ending up as a service type in the service layer. Back then, I attributed that to needing to dependency inject everything all the time where using the new keyword felt like a naughty act. I’ve changed my mind on this - while dependency injection might be a contributing factor, I think there are other reasons why codebases have shifted towards a pattern where the domain model is very slim and the services layer much larger.
I’m guilty of following this pattern; new code almost always tends to match the code that already exists. Bad code doesn’t just rot; it tends to spread too because it’s all too easy to slip into the ’that’s how everyone else has done it, I’ll do it that way too’ kind of thinking, especially when under pressure.
This post aims to look into this typical pattern of having a large services layer with a thin domain model and see what we can do about it.
Anaemic Objects and Services
The term ‘anaemic object’ refers to an object that doesn’t do a lot. It’s usually just a host of public setters and getters. ORMs like entity framework seem to be where this has come from to some extent. They very rarely have any methods on them at all or contain any business logic in them whatsoever.
Following that, you usually have a bunch of services that provide a set of operations on an object. These services generally have no state (except to perhaps store other services injected in the constructor). The services take entity models as parameters in all or some of the methods, these methods then tend to modify the entity object directly, but I’m sure immutable versions exist.
The key point to highlight here is the explicit split between the data and the operations. This makes the code resemble procedural programming much more rather than object orientated programming. The operations are categorised into different types of services that are usually injected into something else, which then carries out a set of steps mixing and matching operations from multiple services to do something.
Procedural programming is simple to understand and easy to read, at first. As complexity increases, the methods in the services tend to become more and more fine-grained so duplication isn’t created, or they’re just flat out duplicated. We know duplication is bad. Making methods more fine-grained on the services reduces encapsulation further and makes the code less maintainable. This is especially the case if each service has a set of unit tests; the tests become so fine-grained and low level that it makes changing or refactoring any part of the code very difficult. Even without tests, the public API of all these services combined will likely be extensive in a significantly sized codebase.
This (anti-)pattern is referred to as a Transaction Script in Martin Fowler’s excellent (they always are) book - Patterns of Enterprise Application Architecture. I also seek to refer to this pattern as that from now on.
It is worth noting that while combining data and logic in a single object is a core principle of object orientated programming, it isn’t always the best way to go. For example, adding all your data access logic into your domain models would be a terrible idea, layering is a far better choice. Some of the common design patterns also separate data and behaviour. There isn’t a golden rule for this, I’m afraid.
The Service Layer
The service layer intends to be a layer between your interface (whether that be a GUI or web API) and the domain model. The problem we’re having with anaemic objects is that our service layer is far too large. The service layer ends up becoming the default place to stick everything.
However, the service layer should not contain business rules at all. It should know nothing or as little as possible about what’s happening the domain, it needs to know enough to call the domain layer, but that’s it. Rather, the services layer should be getting the domain layer to solve the core of the problem but should deal with things like data access. The domain layer shouldn’t know or care about the data access layer. Write code in the domain as if everything was in memory, forget about the database or the TCP socket - they are details that don’t matter to the domain.
Those details do matter to the services layer, though. This is all the services layer should be doing; it should not be providing validation or doing conditional logic for the domain layer.
- Load the data.
- Pass it on to the domain.
- Return the result. Keep it thin.
Moving from Services to Objects
Now we know the service layer should be thin, how the hell do we get rid of some of these services?
Instead of thinking in terms of a set of functions/operations, start thinking about them as things. I could have a MoneyService
with some methods that take a decimal type, and a currency, OR I could have an object called Money
. Money, in this case, would be a special kind of object, a value object. A value object’s equality is based on the value of the object rather than the identity/reference of the object. Some of those methods can be moved to Money
which further allows us to hide the data rather than being forced to make everything public.
You’ll likely find in existing services that certain types of data are almost always passed around together, which is an indication they belong together in an object.
For greenfield projects it’s very easy to jump into the first story and get it across the line with the transaction script pattern. If your project is tiny or more or less a CRUD application, then transaction scripts are probably the right way to go. For domain modelling to work, you need a domain to model. If your project is lasting months then I suspect a domain model likely exists and it’s worth spending the effort trying to come up with a rich domain model. That first story will take longer but you’ll gain that time back later on.
Testing
Which pattern is better to test? I’m very strongly of the opinion that the most important area of an application to test is the domain model. The problem with transaction scripts is that there doesn’t tend to be much cohesion; operations are spread out and categorised into different services all over the place. As the code becomes more complex, more and more services are added, with each one probably having its own access to the data access layer requiring mocks/fakes to be used in the majority of the tests.
A rich domain model, on the other hand, there’s no need to mock anything. You should be able to directly provide the domain layer inputs without needing to mock a data access layer. This is an enormous benefit. A single mock doesn’t usually complicate a test, but many mocks do, so I try and refrain from using them.